Table of contents
- Understanding Single Sign-On and its benefits
- What is Single Sign-On (SSO)?
- How does Single Sign-On work?
- Step-by-Step SSO authentication process
- How this code works?
- The security advantages of Single Sign-On
- The security risk of a compromised SSO
- SAML (Security Assertion Markup Language)
- How OAuth 2.0 works?
- How OpenID Connect works?
- Challenges and limitations of SSO
Understanding Single Sign-On and its benefits
In today’s digital world, managing multiple passwords for different websites and applications can be frustrating and insecure. Single sign-on (SSO) is an authentication method that allows users to access multiple services with a single set of credentials.
By eliminating the need for multiple logins, SSO enhances both security and user experience. This article explores what single sign-on is, how SSO works, and why it’s a crucial component of modern cyber security.
What is Single Sign-On (SSO)?
SSO single sign-on is an authentication solution that enables users to access multiple applications with just one set of login credentials. Instead of logging in separately to each site or service, an SSO system authenticates users once and grants them access to all connected applications.
Example
SSO single sign-on is Google’s authentication system, which allows users to sign into multiple services like Gmail, YouTube, and Google Drive with a single login. Other major providers, such as Microsoft’s Azure AD and Okta, also offer SSO solutions to simplify identity management.
How does Single Sign-On work?
Single sign-on (SSO) authentication relies on a central identity provider to verify user credentials and grant access tokens to multiple applications. This eliminates the need for users to log in multiple times, enhancing both securityand user experience.
In this section, we’ll explore how SSO works, provide a real-world example, and demonstrate an SSO implementation with code.
Step-by-Step SSO authentication process
To better understand how SSO single sign-on works, let’s walk through a typical authentication flow with a real-world example:
User logs in once
- The user tries to access an SSO-enabled website or application.
- The system redirects the user to an identity provider (IdP) like Okta, Microsoft Entra ID, or Google Identity Platform.
Identity provider authenticates the user
- The user enters their credentials (username and password).
- If multi-factor authentication (MFA) is enabled, an additional security step (e.g., OTP, biometrics) is required.
- Once verified, the identity provider generates an authentication token.
Access to multiple applications without additional logins
- The identity provider redirects the user back to the requested application with an authentication token.
- The application validates the token and grants access without requiring another login.
Active session until logout
- The user can navigate between multiple websites and applications using SSO without re-authenticating.
- The session remains valid until the user logs out or the token expires.
Practical example with OpenID Connect and OAuth 2.0
To show a real implementation of SSO, let’s consider a web application that uses OpenID Connect (OIDC) and OAuth 2.0 to authenticate users via Google Identity Platform.
Setting up the Identity Provider (Google Identity Platform)
First, you need to register your application with Google Cloud Console to obtain OAuth 2.0 API credentials.
- Create a new project in Google Cloud.
- Navigate to “Credentials” → “Create Credentials” → “OAuth Client ID”.
- Configure the Redirect URI (e.g., http://localhost:3000/callback).
- Copy the Client ID and Client Secret.
Implementing SSO in a Node.js application
We create a server in Node.js that handles SSO authentication using Passport.js with OAuth 2.0.
Install the necessary dependencies:
sh
npm install express passport passport-google-oauth20 express-session dotenv
Code for Setting Up Google SSO in Node.js
javascript
require('dotenv').config();
const express = require('express');
const session = require('express-session');
const passport = require('passport');
const GoogleStrategy = require('passport-google-oauth20').Strategy;
const app = express();
// Configure session middleware
app.use(session({
secret: 'superSecretKey',
resave: false,
saveUninitialized: true
}));
// Initialize Passport.js
app.use(passport.initialize());
app.use(passport.session());
// Configure Google OAuth 2.0 strategy
passport.use(new GoogleStrategy({
clientID: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
callbackURL: "/auth/google/callback"
}, (accessToken, refreshToken, profile, done) => {
return done(null, profile);
}));
// Serialize user session
passport.serializeUser((user, done) => done(null, user));
passport.deserializeUser((obj, done) => done(null, obj));
// Route to initiate Google SSO
app.get('/auth/google', passport.authenticate('google', { scope: ['profile', 'email'] }));
// Google OAuth callback route
app.get('/auth/google/callback',
passport.authenticate('google', { failureRedirect: '/' }),
(req, res) => {
res.redirect('/dashboard');
}
);
// Protected dashboard route
app.get('/dashboard', (req, res) => {
if (!req.isAuthenticated()) {
return res.redirect('/');
}
res.send(`Welcome, ${req.user.displayName}`);
});
// Logout route
app.get('/logout', (req, res) => {
req.logout(() => {
res.redirect('/');
});
});
app.listen(3000, () => console.log('Server running at http://localhost:3000'));
How this code works?
- Google OAuth 2.0 is configured using the Client ID and Client Secret from Google Cloud.
- Passport.js handles authentication using GoogleStrategy.
- Users are redirected to Google when they access /auth/google.
- Once authenticated, Google redirects them back to /auth/google/callback.
- If successful, the user is granted access to the dashboard.
- The authentication token is stored in a session, allowing users to remain logged in until they log out.
Example of a JWT Token issued by Google SSO
When a user logs in via Google SSO, an OAuth 2.0 token is generated. The token is in JWT (JSON Web Token) format, which can be decoded to reveal information about the authenticated user.
Here’s an example JWT payload:
json
{
"alg": "RS256",
"kid": "abcd1234",
"typ": "JWT"
}
{
"iss": "https://accounts.google.com",
"sub": "1234567890",
"email": "user@example.com",
"name": "John Doe",
"iat": 1712345678,
"exp": 1712349278
}
"sub": Unique user ID.
"email": The authenticated user’s email.
"exp": Expiration timestamp.
We have seen how Single Sign-On works, analyzing the process with a practical example and code. Implementing an SSO system with OAuth 2.0 and OpenID Connect allows users to access multiple applications with a single login, improving security and user experience.
For further reading, check out:
- Google Identity Platform Docs
- OAuth 2.0 Guide
The security advantages of Single Sign-On
Adopting SSO solutions significantly improves cyber security by reducing risks associated with password management and access control. Below, we explore the key security advantages of SSO single sign-on with practical examples.
Reducing weak password usage
One of the biggest security vulnerabilities is password fatigue, where users struggle to remember multiple passwords and resort to weak or reused credentials.
Example
A multinational corporation with thousands of employees previously required different credentials for each internal system (email, HR portal, document storage, etc.). Employees often used weak passwords or repeated the same password across systems, increasing the risk of data breaches.
By implementing SSO with Microsoft Entra ID, employees now authenticate once and gain access to all corporate applications. This has:
- Eliminated the need to manage multiple passwords;
- Reduced the risk of weak or repeated credentials;
- Improved security without compromising productivity.
Lower risk of phishing attacks
Phishing attacks often trick users into entering their credentials on fake login pages. Since SSO requires users to log in less frequently, the opportunity for phishing attacks is greatly reduced.
Example
A large bank noticed an increase in phishing email attempts where attackers sent fake login requests to employees, leading to unauthorized access to sensitive financial records.
After implementing Okta SSO, the bank has:
- Eliminated the risk of credential-based phishing, as users no longer have to enter passwords directly into services;
- Integrated passwordless authentication with security keys, preventing the use of stolen credentials.
Integration with Multi-Factor Authentication (MFA)
Although SSO reduces login frequency, it must be paired with MFA to prevent unauthorized access if a single credential is compromised.
Example: cloud-based SaaS security
A technology startup relies on multiple cloud-based SaaS applications, such as Slack, Salesforce, and AWS. Without SSO, employees logged into each application separately, and many neglected to enable MFA on all services.
After implementing Google Workspace SSO with mandatory MFA, the system now requires:
- One login for all applications;
- Biometric authentication or OTP code to verify identity;
- Increased protection against unauthorized access.
Even if a hacker were to obtain a password, he could not gain access without completing the second level of verification.
Centralized access control and account management
With SSO, IT administrators can manage access to enterprise services from a single platform, making it easier to revoke credentials when needed.
Example: healthcare industry & HIPAA compliance
A hospital network manages thousands of doctors, nurses, and administrative staff who need access to electronic medical records (EMRs). Before SSO, each user had multiple separate accounts, making it difficult to monitor access and revoke permissions in case of layoffs or transfers.
With SSO OneLogin, IT teams can now:
- Automatically revoke access when an employee leaves, reducing insider threats.
- Assign role-based access, ensuring that only authorized personnel can view patient records.
- Generate access reports, improving GDPR and HIPAA compliance.
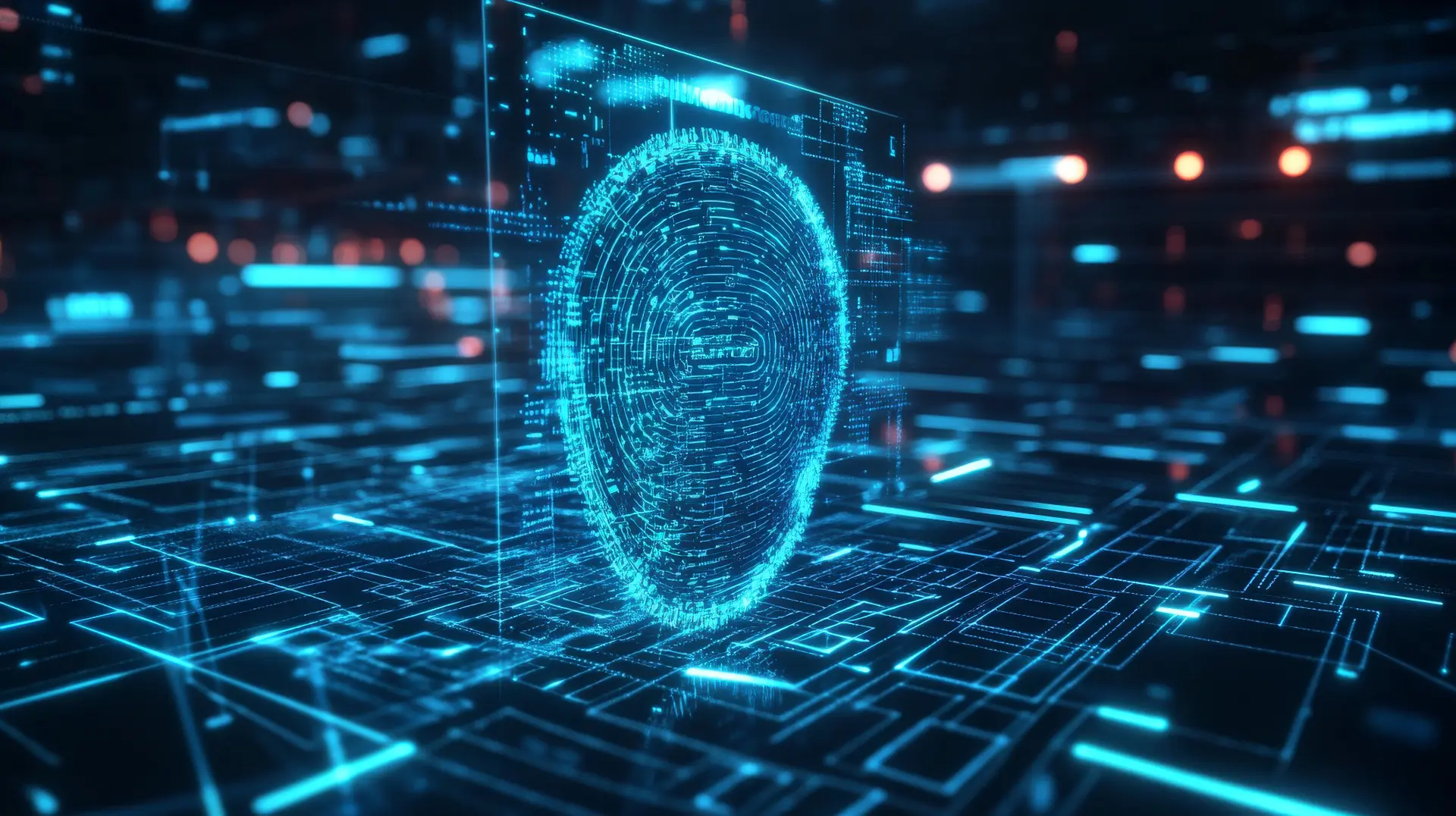
The security risk of a compromised SSO
While SSO improves security, it also creates a single point of failure: if the SSO provider is breached, all connected applications could be compromised.
Example: a large-scale SSO provider attack
In 2022, a major SSO provider was targeted by hackers, potentially exposing access to thousands of companies. The attack exploited a weakly secured admin account, allowing attackers to gain access to the provider’s authentication systems.
Companies relying solely on SSO without MFA were left vulnerable, as attackers could generate valid session tokens to access corporate applications.
How to mitigate this risk?
- Always enforce multi-factor authentication (MFA) to prevent unauthorized access even if SSO credentials are leaked.
- Monitor access logs for unusual login attempts, especially from unknown devices or locations.
- Adopt a Zero Trust approach, ensuring continuous verification of user identity and device security.
Common Single Sign-On protocols
SSO single sign-on systems rely on authentication protocols to enable secure, seamless user access across multiple applications and platforms.
These protocols act as the foundation for identity verification, ensuring that users can log in once and access multiple services without repeatedly entering credentials.
The most widely used SSO authentication protocols include SAML, OAuth 2.0, and OpenID Connect (OIDC). Each serves a specific function and is suited for different use cases. Let us delve into how they work and see some practical examples of their application.
SAML (Security Assertion Markup Language)
SAML (Security Assertion Markup Language) is an XML-based authentication protocol that enables secure user authentication via identity providers (IdPs). Companies and institutions use SAML to enable users to access multiple applications with a single authentication, without having to manage separate credentials.
How SAML works
SAML authentication follows a federated identity model, where a user logs in once through an identity provider (IdP), and their credentials are verified and passed to other applications (service providers).
- User attempts to access a service provider (SP), such as Salesforce or Google Workspace.
- The SP redirects the user to an identity provider (IdP) like Okta or Microsoft Entra ID for authentication.
- The IdP verifies the user’s credentials and generates a SAML assertion (a signed XML document).
- The SAML assertion is sent back to the service provider, confirming the user’s identity.
- The user is granted access without needing to log in again.
Example: SAML in enterprise IT
A multinational company uses SAML-based SSO with Okta to allow employees to access multiple work applications with a single login. Once an employee logs into Okta, they can seamlessly access their email (Microsoft Outlook), document storage (Google Drive), and HR portal (Workday) without entering credentials multiple times.
Advantages of SAML
- High security through encryption and digital signature of assertions.
- Reduced password management, improving user experience.
- Ideal for enterprise and enterprise environments with multiple interconnected services.
OAuth 2.0: secure API authentication
OAuth 2.0 is an authorization framework that enables applications to gain secure access to APIs without having to manage user credentials directly.
How OAuth 2.0 works?
Instead of sharing username and password, OAuth 2.0 issues access tokens that applications use to interact with APIs securely.
- The user logs into a third-party application (e.g., a mobile app requesting access to Google Drive).
- The app redirects the user to the authorization server (e.g., Google).
- The user grants permission for the app to access their data.
- Google issues an access token to the app, allowing it to retrieve the requested data.
- The app uses the token to make API calls, without ever seeing the user’s password.
Example: OAuth 2.0 in social media authentication
A news site allows users to register and log in using their Google or Facebook account, instead of creating a new account with email and password. OAuth 2.0 allows the site to authenticate the user without ever asking for their credentials, instead using an access token provided by Google or Facebook.
Advantages of OAuth 2.0
- Enhances security
Applications never see or store user passwords. - Enables seamless third-party integrations
Used in mobile apps, cloud services, and APIs. - Supports fine-grained access control
Users can grant specific permissions (e.g., read-only access to data).
OpenID Connect (OIDC): authentication built on OAuth 2.0
OpenID Connect (OIDC) is an authentication protocol built on top of OAuth 2.0, designed for user identity verification. While OAuth 2.0 focuses on authorization (granting permissions to apps), OIDC ensures secure authentication by providing identity tokens (ID tokens).
How OpenID Connect works?
OIDC follows a process similar to OAuth 2.0, but instead of only issuing an access token, it also issues an ID token that contains user identity details.
- The user attempts to access a website using his or her Google account.
- The site redirects the user to Google for authentication.
- Google verifies the user’s identity and generates an ID token, containing details such as name, email, and profile photo.
- The site receives the token and confirms the user’s identity without the need for additional passwords.
Example: OpenID Connect in Google Login
When you sign in to a new online service using the “Sign in with Google” button, the website uses OpenID Connect to obtain verified information about your identity, without directly storing your password.
Advantages of OpenID Connect
- Adds authentication to OAuth 2.0, enabling secure user logins.
- Widely supported by identity providers, including Google, Microsoft, and Okta.
- Reduces dependence on passwords, improving security and usability.
Comparing SAML, OAuth 2.0, and OpenID Connect
Protocol | Purpose | Best used for | Example use case |
SAML | Authentication via identity providers | Enterprise SSO | Large organizations using Okta or Microsoft Entra ID for employee logins |
OAuth 2.0 | Authorization for third-party applications | API access and mobile apps | Allowing apps to connect to Google Drive, Facebook, or Twitter without sharing passwords |
OIDC | Authentication built on OAuth 2.0 | User authentication | Logging into websites using a Google or Microsoft account |
Choosing the right SSO protocol
- Use SAML for enterprise SSO in large organizations that need secure employee authentication across multiple applications.
- Use OAuth 2.0 when developing applications that require secure API access, such as mobile apps and cloud services.
- Use OpenID Connect (OIDC) for modern web and mobile authentication, ensuring secure user logins with identity verification.
By implementing the most suitable protocol, companies can improve security, reduce credential management and provide a smoother user experience.
SSO use cases in different industries
Many industries leverage SSO authentication to streamline user access and enhance security. Some key sectors include:
- Enterprise IT
Large organizations use SSO solutions like Microsoft Entra ID and Okta to enable secure access to business applications.
- Healthcare
Hospitals implement SSO to ensure doctors and nurses can quickly access electronic medical records without multiple logins.
- E-commerce
Online retailers allow customers to log in via Google or Facebook SSO, improving user convenience.
- Education
Universities use SSO sign on solutions to give students and faculty seamless access to learning management systems.
Challenges and limitations of SSO
Despite its benefits, SSO authentication has some challenges. The biggest concern is that if the main SSO account is compromised, all linked accounts are at risk. Additionally, implementing SSO solutions can be complex, requiring integration with existing IT infrastructure and third-party identity providers.
To mitigate these risks, organizations often use SSO combined with multi-factor authentication and robust identity management policies.
Conclusion: is SSO right for you?
For businesses and organizations looking to enhance security, improve user experience, and streamline authentication, SSO single sign-on is a powerful solution. However, it should be implemented with MFA and strong access controls to ensure maximum security. As the digital landscape evolves, SSO solutions will continue to play a critical role in modern cyber security strategies.
Questions and answers
- What is single sign-on (SSO)?
Single sign-on (SSO) is an authentication method that allows users to access multiple applications with a single set of login credentials. - How does single sign-on work?
SSO works by using an identity provider to authenticate a user once and grant access to multiple connected applications without requiring additional logins. - What are the benefits of using SSO?
SSO solutions reduce password fatigue, enhance security, support multi-factor authentication, and simplify user access management. - Is SSO safe?
Yes, when properly implemented with multi-factor authentication (MFA) and strong security policies, SSO authentication is highly secure. - What are the risks of single sign-on?
The primary risk is that if the SSO provider is compromised, all connected accounts could be at risk. MFA helps mitigate this issue. - Which industries use SSO the most?
SSO solutions are widely used in enterprise IT, healthcare, education, e-commerce, and financial services for secure and seamless authentication. - What are some common SSO providers?
Popular SSO providers include Okta, Microsoft Entra ID (Azure AD), Google Identity Platform, and OneLogin. - What is the difference between SSO and MFA?
SSO single sign-on enables access to multiple applications with one login, while multi-factor authentication (MFA) adds an extra security layer by requiring additional verification. - Does Google offer SSO?
Yes, Google’s SSO authentication system allows users to log in once and access multiple Google services like Gmail, YouTube, and Google Drive. - Can small businesses use SSO?
Yes, many cloud-based SSO solutions cater to small businesses, providing cost-effective and scalable authentication methods.